I’ve always preferred camel caps to underscores when it comes to naming conventions, but I’ve never really known why. Recently I’ve been writing a lot of Python, where the standard is to use underscores for most names, and now I realise why I don’t like underscores. I hope to make my argument for camel caps objective — or, failing that, at least better than the mouth-frothing, religious reactions you normally get in these “X is better than Y” type of discussions.
Why camelCaps Are Superior To_Underscores
25 Jul, 2012 — Category: Coding Style/Conventions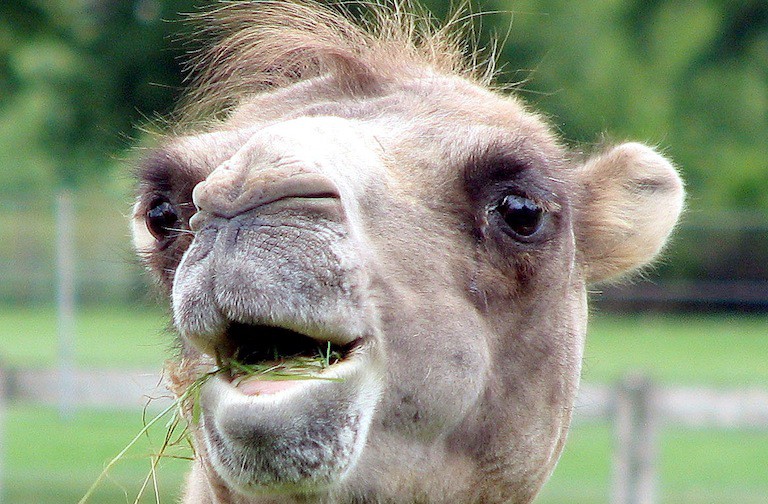