In this article, we will be adding directional lights, spotlights, and allowing for multiple lights instead of just one. This is the final article on lighting – at least for a while.
Modern OpenGL 08 – Even More Lighting: Directional Lights, Spotlights, & Multiple Lights
01 Nov, 2014 — Category: Modern OpenGL Series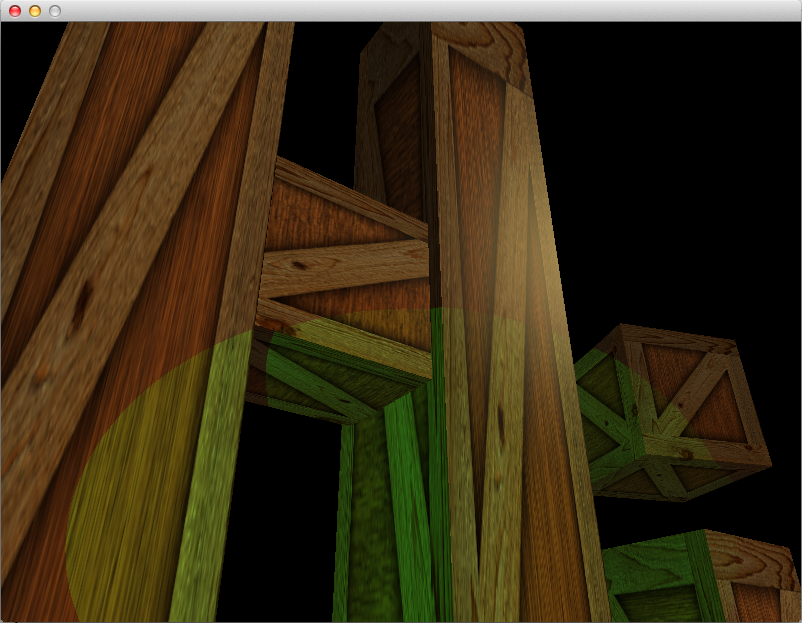